23 Apr 2014
大部分pc到客户端引流都采用二维码扫描的方式,扫码这里就不说了,关键说一下如何从扫码到唤起客户端,暂时仅包括android和ios两大平台。
处理逻辑
- 扫码到一个http页面
- 页面上写javascript通过useragent判断当前平台android/iphone/ipad;
- create一个隐藏的frame,设置他的src为应用注册的schema来唤起客户端,比如淘宝主客就是taobao://;
- 如果用户没有安装客户端,再通过settimeout引导到客户端下载页;
- 这里要特别注意android和ios一个最大的区别是android唤起的时间要比ios慢许多;比如ios只需要200ms就可以判断唤起,但是android起码要600ms,所以引导到客户端下载页的timeout要有所区别;
代码示例
var noClientCallback = function(){
window.location = “http://2.taobao.com/m.html”;
};
(function(){
var outTime = (navigator.userAgent.match(/android/i))?600:200;
var goUrl = “taobao://wnwebview?url=http://www.taobao.com/”;
var e_frame = document.createElement(‘frame’);
e_frame.setAttribute(‘id’, ‘J_smartFrame’);
e_frame.style.cssText = ‘display:none’;
document.body.appendChild(e_frame);
e_frame.setAttribute(‘src’,goUrl);
window.setTimeout(function(){
if(e_frame){
document.body.removeChild(e_frame);
}
noClientCallback();
},outTime);
})();
Android Update
最近发现部分android手机不能正常唤起客户端,主要原因google有解释:https://developer.chrome.com/multidevice/android/intents
也就是说现在Android的chrome下(25及以后的版本),上面的隐藏iframe方式不能正常生效了,唤起客户端需要这么写:
window.location = ‘intent://item/#Intent;scheme=taobao;package=com.taobao;end’;
如果还需要带一些参数的话,可以写在item后面
window.location = ‘intent://item?id=12345678/#Intent;scheme=taobao;package=com.taobao;end’;
另外如果还有唤起客户端的需要,建议看看这个repo:https://github.com/miaojing/redirectToNative
以及他的demo:http://gallery.kissyui.com/redirectToNative/1.2/demo/index.html
23 Apr 2014
Class & id 的定义
首先看看在objective-c中对于class和object的定义,这个在/usr/include/objc/objc.h 和 runtime.h中可以找到:
typedef struct objc_class *Class;
typedef struct objc_object {
Class isa;
} *id;
class是一个objc_class的指针,id(也就是对象)是一个指向objc_object结构体的指针,所以每个对象都有一个纸箱class(objc_class)的isa指针;
objc_class 的定义
那么objc_class又是怎样的呢?
struct objc_class
{
struct objc_class* isa;
struct objc_class* super_class;
const char* name;
long version;
long info;
long instance_size;
struct objc_ivar_list* ivars;
struct objc_method_list** methodLists;
struct objc_cache* cache;
struct objc_protocol_list* protocols;
};
可以看到class又有一个指向class的isa指针,这个class就是我们俗称的metaclass,也就是类对象的isa指向的就是metaclass;
metaclass跟class的区别在于metaclass存储类static开头的方法和static开头的成员(+开头的方法);class存储类的实例方法和实例变量(-开头的);
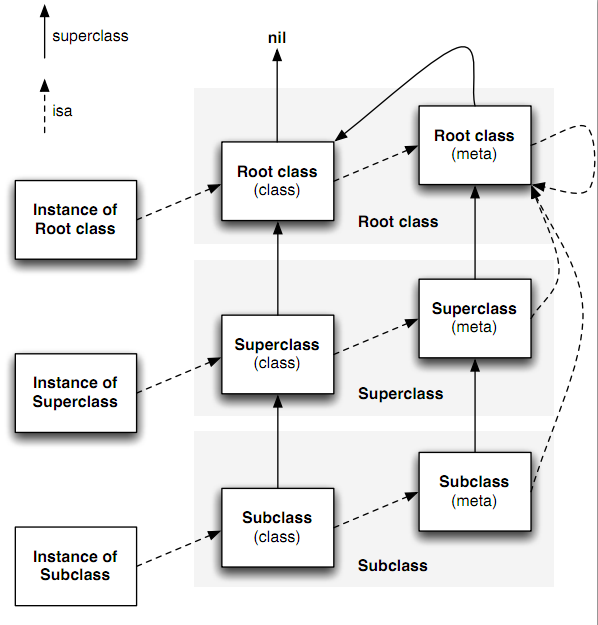
- 类的实例对象的isa指向类,类的isa指向类的metaclass,类的metaclass指向基类的metaclass
- 类的superclass指向父类,类的metaclass的superclass指向该类的superclassmetaclass
- 基类的superclass指向nil,基类的metaclass指向基类自己
说完了isa和superclass的关系,我们再说一下objc_class的其他属性
- name:一个c字符串,指向类的名称。我们可以在运行期,通过这个名称查找到该类(通过:id objc_getClass(const char *aClassName))或者该类的metaclass(id objc_getMetaClass(const char *aClassName))
- version:类的版本信息,默认初始化为0.我们可以在运行期对其进行修改(class_setVersion)或后去(class_getVersion)
- info:标示类的类型,比如是metaclass还是class
- instance_size:该类的实例变量大小
- ivars:实例变量
- methodLists:如果上面info说明当前是class,这里存储实例方法,如果是metaclass,这里存储类额静态方法
- cache:指向objc_cache的指针,用于对方法的缓存
- protocols:指向objc_protocol_list的指针,存储该类要遵守的协议
内容参考自:http://www.cnblogs.com/kesalin/archive/2012/01/19/objc_class_object.html
23 Apr 2014
原文:http://blog.logichigh.com/2011/03/16/when-does-layoutsubviews-get-called/
So the results is below:
- init does not cause layoutSubviews to be called (duh)
- addSubview causes layoutSubviews to be called on the view being added, the view it’s being added to (target view), and all the subviews of the target view
- setFrame intelligently calls layoutSubviews on the view having it’s frame set only if the size parameter of the frame is different
- scrolling a UIScrollView causes layoutSubviews to be called on the scrollView, and it’s superview
- rotating a device only calls layoutSubview on the parent view (the responding viewControllers primary view)
- removeFromSuperview – layoutSubviews is called on superview only (not show in table)
翻译如下
- init不会引起layoutSubviews的调用;
- addSubview会引起父view以及该父view所有子view的layoutSubviews方法的调用;
- setFrame会在frame发生变化的时候引起layoutSubview的调用;
- 滚动一个UIScrollview会引起该UIScrollview以及其父view的layoutSubviews的调用;
- 滚动设备会引起当前viewController的view的layoutSubviews调用;
- removeFromSuperview会调用父view的layoutSubviews;
23 Apr 2014
In a non-ARC environment this Rules to remember
- You have ownership of any objects you create.
- You can take ownership of an object using retain.
- When no longer needed, you must relinquish ownership of an object you own.
- You must not relinquish ownership of an object you don’t own.
Take ownership of a object in this methods which name likes
- alloc
- new
- copy
- mutableCopy
- and “retain” also take the ownship
In ARC , follow below rules
- Forget about using retain, release, retainCount, and autorelease.
- Forget about using NSAllocateObject and NSDeallocateObject.
- Follow the naming rule for methods related to object creation.
- Forget about calling dealloc explicitly.
- Use @autoreleasepool instead of NSAutoreleasePool.
- Forget about using Zone (NSZone).
- Object type variables can’t be members of struct or union in C language.(__bridge __bridge_retain __bridge_transfer)
- ‘id’ and ‘void*’ have to be cast explicitly.
the property qualifier means
Property modifier |
Ownership qualifier |
assign |
__unsafe_unretained |
copy |
__strong(note:new copied object is assigned.)__strong |
retain |
__strong |
strong |
__strong |
weak |
__weak |
unsafe_unretained |
__unsafe_unretained |
23 Apr 2014
In a non-ARC environment this Rules to remember
- You have ownership of any objects you create.
- You can take ownership of an object using retain.
- When no longer needed, you must relinquish ownership of an object you own.
- You must not relinquish ownership of an object you don’t own.
Take ownership of a object in this methods which name likes
- alloc
- new
- copy
- mutableCopy
- and “retain” also take the ownship
In ARC , follow below rules
- Forget about using retain, release, retainCount, and autorelease.
- Forget about using NSAllocateObject and NSDeallocateObject.
- Follow the naming rule for methods related to object creation.
- Forget about calling dealloc explicitly.
- Use @autoreleasepool instead of NSAutoreleasePool.
- Forget about using Zone (NSZone).
- Object type variables can’t be members of struct or union in C language.(__bridge __bridge_retain __bridge_transfer)
- ‘id’ and ‘void*’ have to be cast explicitly.
the property qualifier means
Property modifier |
Ownership qualifier |
assign |
__unsafe_unretained |
copy |
__strong(note:new copied object is assigned.)__strong |
retain |
__strong |
strong |
__strong |
weak |
__weak |
unsafe_unretained |
__unsafe_unretained |